In this post, we will understand the concept of Svelte two way binding with examples.
Basically, two-way binding automatically detects when an attribute on a particular element changes and reflects the same change in the local variable inside your component. In other words, two-way binding keeps a close integration between the model and view.
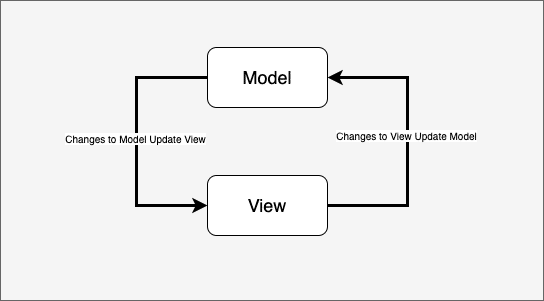
With the advent of frameworks such as React, two-way binding became less popular. In fact, React did not support two-way binding. It was mostly considered as error-prone and hence fell out of favour.
However, Svelte comes with first-class support for two-way binding.
In case you are new to Svelte, I will recommend you to go through this walkthrough of a typical Svelte application.
1 – What is One-Way Binding?
Before we see two-way binding, let’s try to understand how a typical one-way binding works.
In a typical one-way binding approach, we set the value for a variable in the normal HTML way. Changes to the value are tracked using event handlers.
See below example:
<script>
export let name = "Fantasy";
function changeName(event) {
const updatedName = event.target.value;
name = updatedName
}
</script>
<p>Welcome to the {name} Library</p>
<input on:input="{changeName}"/>
Here, we have the name variable. We use it in the markup for the welcome message. However, we also have an input field where we use the on:input event to fire a function changeName(). Basically, this function receives the event and updates the value of name on every keystroke by the user. This also updates the DOM with the updated value of name. In other words, there is a uni-directional flow of data in our application where we update the welcome message on every change to the name field.
This is also known as one-way binding.
2 – Svelte Two Way Binding
Two-way binding works in a similar manner. However, the syntax is quite minimal and the framework adds the necessary boilerplate code behind the scenes.
Let’s see how we do it in Svelte.
<script>
export let name = "Fantasy";
function changeName(event) {
const updatedName = event.target.value;
name = updatedName
}
</script>
<p>Welcome to the {name} Library</p>
<input bind:value="{name}"/>
Here, we use the bind keyword. Svelte understands this keyword. Here, we specify that we wish to bind the value of the input element to the name attribute in the component. The data flows into the input when user types in the browser and with every keystroke, the name is also updated.
In other words, we have two-way binding.
Conclusion
With this we have successfully understood how Svelte two-way binding works. It is a really cool feature of Svelte that can help developers avoid a bunch of boilerplate code. However, it should be used only when absolutely necessary.
If you have any comments or queries about this post, please mention in the comments section below.
0 Comments