Forms are an integral part of any web application. We use forms all the time to collect data from the users. Since it is possible that users may enter invalid data from our application perspective, it is important to implement form validation. However, Svelte does not have an out-of-box form validation feature. This naturally leads to looking for libraries that can make our job easy. However, that also makes things more complicated. But can we actually perform Svelte Custom Form Validation without using any External Libraries?
We can perform custom form validation in Svelte very easily. There is no need to use any external libraries especially for simple validations. We can also implement an interactive form validation approach where user gets continuous feedback on whether the form is valid or not.
In this post, we will be building a small Svelte form validation example. We will also be taking care of displaying error messages for our form fields and disable form submission in case the form is not valid.
1 – Create a Svelte Form Layout
We will first create a basic form layout in a Svelte component.
See below:
<form on:submit|preventDefault={addBookHandler}>
<div class="form-field">
<label for="title">Book Title:</label>
<input type="text" id="title" bind:value={fields.title} on:input={handleInput}>
<div class="error">{ errors.title }</div>
</div>
<div class="form-field">
<label for="author">Author:</label>
<input type="text" id="title" bind:value={fields.author} on:input={handleInput}>
<div class="error">{ errors.author }</div>
</div>
<Button buttonType="secondary" disabled={!valid}>Add Book</Button>
</form>
This form basically collects information about books. We have two fields – title and author. Apart from the label and input HTML elements, we also have a div with CSS class error. This is where we will display error messages for the input form data.
For every field, we listen to the on:input event and call the handler function handleInput(). This is important for interactive validation of the form as the user enters data. Basically, we are performing Svelte form validation without submit of the form.
Also, we use the on:submit directive and attach it to handler function addBookHandler(). We also use a custom button component. However, you can also use a normal button.
If interested, you can read more about form-handling in Svelte.
2 – Implement Svelte Custom Form Validation
Next step is to actually perform the validation. This is done in the script section of our Svelte component.
See below:
<script>
import Button from '../shared/Button.svelte';
let fields = { title: '', author: '' };
let errors = { title: '', author: ''};
let valid = false;
const addBookHandler = () => {
if (valid) {
console.log('valid', fields)
}
}
const handleInput = (event) => {
valid = false
if (fields.title.trim().length < 1) {
valid = false;
errors.title = 'Book Title should have at least 1 character';
}else {
valid = true
errors.title = '';
}
if (fields.author.trim().length < 1) {
valid = false;
errors.author = 'The Name of the Author should have at least 1 character';
}else {
valid = true
errors.author = '';
}
}
</script>
We validate the user entries in the form fields within the handleInput() function. Basically, we check if the title and author are more than 1 character long. If not, we update the errors object with a suitable message. The fields object stores the actual value of the form field.
Depending on your application requirements, you can have as many checks and error messages. The valid variable keeps track of the form’s validity at any given point of time.
Once the user submits the form, we call the addBookHandler() method. In this example, we simply do a console log of the data. In a real application, this will be the place where you can make a call to the store or API to actually store the data.
3 – Styling Form Validation Error Messages
We also use some simple CSS styling to make our form look good.
<style>
form {
width: 400px;
margin: 0 auto;
text-align: center
}
.form-field {
margin: 18px auto;
}
input {
width: 100%;
border-radius: 6px;
}
label{
margin: 10px auto;
text-align: left;
}
.error {
font-weight: bold;
font-size: 12px;
color: red;
}
</style>
Note how the CSS class error uses different font size and colour to emphasize to the user that something is wrong in the data they have entered.
In case you are interested to know more about styling in Svelte, do check out our detailed post about CSS in Svelte.
Below is the complete form component code for reference.
<script>
import Button from '../shared/Button.svelte';
let fields = { title: '', author: '' };
let errors = { title: '', author: ''};
let valid = false;
const addBookHandler = () => {
if (valid) {
console.log('valid', fields)
}
}
const handleInput = (event) => {
valid = false
if (fields.title.trim().length < 1) {
valid = false;
errors.title = 'Book Title should have at least 1 character';
}else {
valid = true
errors.title = '';
}
if (fields.author.trim().length < 1) {
valid = false;
errors.author = 'The Name of the Author should have at least 1 character';
}else {
valid = true
errors.author = '';
}
}
</script>
<form on:submit|preventDefault={addBookHandler}>
<div class="form-field">
<label for="title">Book Title:</label>
<input type="text" id="title" bind:value={fields.title} on:input={handleInput}>
<div class="error">{ errors.title }</div>
</div>
<div class="form-field">
<label for="author">Author:</label>
<input type="text" id="title" bind:value={fields.author} on:input={handleInput}>
<div class="error">{ errors.author }</div>
</div>
<Button buttonType="secondary" disabled={!valid}>Add Book</Button>
</form>
<style>
form {
width: 400px;
margin: 0 auto;
text-align: center
}
.form-field {
margin: 18px auto;
}
input {
width: 100%;
border-radius: 6px;
}
label{
margin: 10px auto;
text-align: left;
}
.error {
font-weight: bold;
font-size: 12px;
color: red;
}
</style>
This is how the Svelte form validation with error messages looks like in action when we start the application.
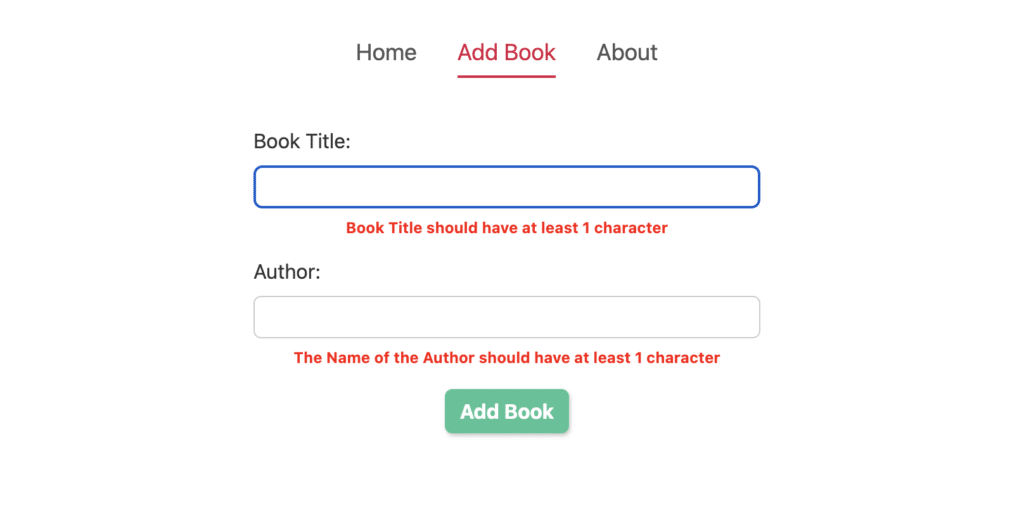
Once the user starts to enter some data and it does not satisfy the checks, we see the error messages pop up below the respective fields.
Conclusion
With this, we have successfully learnt how to setup custom form validation in Svelte. And we did this without using any libraries or frameworks.
Want to learn more about building customizable components in Svelte? Check out this post on creating a custom reusable tab component in Svelte like the one in the screenshot.
If you have any comments or queries about this post, please feel free to mention them in the comments section below.
0 Comments