Components are the building blocks for large applications. A good component design can help us build more modular applications and maintain them better for future use. In this post, we will look at a Vue 3 component example.
Basically, components allow us to split the overall user interface into independent and reusable pieces. Each piece can have its own isolated state and functionality. You could think of the UI as a tree of components similar to how we actually visualize the DOM.
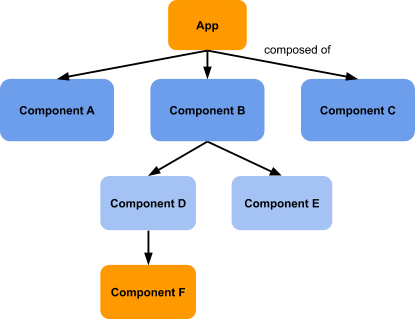
While the component hierarchy looks similar to native HTML, but VueJS implements its own component model. This model allows us to encapsulate content and logic in a coherent way.
If you are new to VueJS, I will suggest starting with the post on Vue 3 application bootstrap.
1 – Defining a Vue 3 Component Example
Each Vue component is defined using a dedicated .vue
file. This is also known as SFC or Single File Component.
See below example of a sample component in VueJS.
<script setup>
import { ref } from 'vue'
const count = ref(0)
</script>
<template>
<button @click="count++">
Click Counter: {{ count }}
</button>
</template>
As you can see, this is a very simple component. It has a counter variable declared using ref()
function. This brings the VueJS reactivity into the picture. Basically, any change to the count
will trigger a re-render of the DOM.
Within the template
section, we have a button and a click handler that simply increments the count
. Within the button text, we display the counter value at the given point of time.
2 – Using the VueJS Component
The whole point of having components is to increase the reusability of your application. Therefore, it becomes important to understand how to actually use the component in another component.
See the below example:
<script setup>
import Counter from './Counter.vue'
</script>
<template>
<h1>Button Components with Counter</h1>
<Counter />
<Counter />
<Counter />
</template>
As you can see, we first import the Counter
component into the App
component. This is done using the import statement.
Then, we can simply use the Counter
component in the template
section. For demo purpose, we are using it multiple times.
Important point to note here is that each iteration of the Counter
component is a different instance. In other words, each instance has its own value for the count
. Basically, every time we use a component, it creates a new instance.
We can also import multiple components and use them within our main component if required. You can think of them as lego blocks where you can join blocks to build complex structures.
Conclusion
This concludes our first venture into a Vue 3 Component Example. Components are a key aspect of VueJS and many other modern web frameworks.
An important aspect to consider is the correct size of a component and how to break up a functionality into multiple components to make it easy to use.
Want to use make your components more dynamic? Check out this guide on how to use Vue 3 Component Props.
If you have any comments or queries about this post, please feel free to mention them in the comments section below.
0 Comments