JSX stands for Javascript XML. Basically, React JSX is the method using which you can describe the UI in a React application.
The philosophy behind React is that the rendering logic or the presentation logic is tightly coupled with other UI logic. In other words, various operations such as handling events, making API Calls and preparing data for display can affect the user interface.
Therefore, React provides separation of concern by means of loosely coupled units known as Components. Basically, you can consider your DOM or Document Object Model to be built using several components. And each of those components is described by its own JSX.
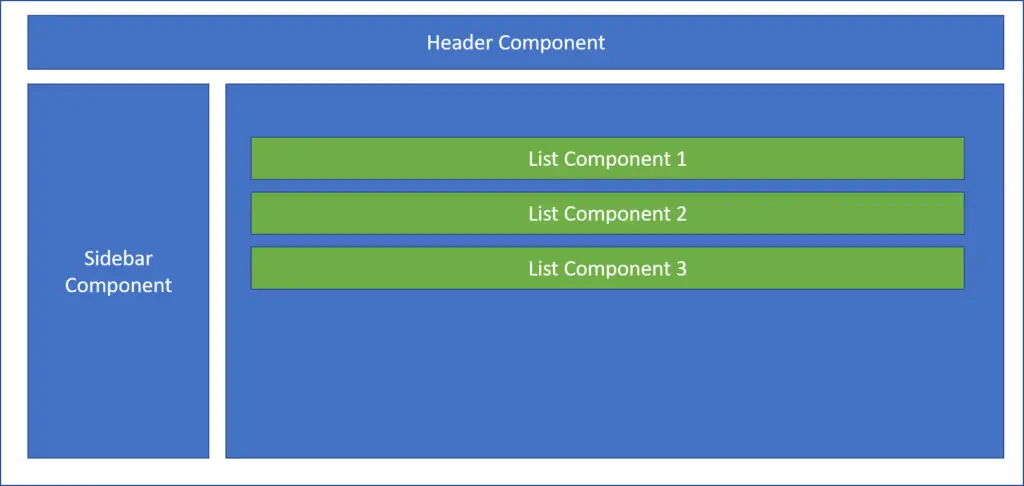
1 – React JSX Example
As discussed, JSX is just a way of describing the interface. And hence, it can also be a simple element displaying some text. See below:
const element = <h1>Hello world from React</h1>
Here, we simply have a constant storing an HTML tag with some text. And this is a perfectly valid piece of JSX.
2 – Expressions in React JSX
JSX can also contain expressions. In other words, any valid expression we place within curly braces inside a JSX will be evaluated and rendered. Below is an example:
const element = <h1>{5 + 2}</h1>
Here, 5 + 2 is a valid expression. And hence, we can use it inside the JSX with ease.
We could also do something like below:
const name = 'Progressive Web Ninja'
const element = <h1>{name}</h1>
Here, we declare a constant called name and assign it a value. Then, using the JSX expression syntax, we inject it within the h1 tag.
To add, we can also inject functions within the JSX just like expressions. See below example:
function joinName(siteName) {
return user.firstName + ' ' + user.lastName;
}
const siteName = {
firstName: 'Progressive',
lastName: 'Ninja'
};
const element = <h1>{joinName(siteName)}</h1>
As you can see, things are quite flexible when it comes to JSX.
3 – JSX is an Expression
JSX gets eventually converted to Javascript function calls. They are evaluated as Javascript objects. Hence, we can treat them also as expressions.
Basically, this means that we can use JSX in conditional statements as below:
function greeting(siteName) {
if (siteName) {
return <h1>Hello, {joinName(user)}!</h1>;
}
return <h1>Hello, I don't know you.</h1>;
}
Based on the evaluation of the if-condition, we return different pieces of JSX as output of the greeting() function. The greeting() function can then be embedded into another JSX just like a normal expression.
This provides a great amount of flexibility while building complex applications.
4 – JSX Attributes
We can also pass attributes to our JSX elements. See below example:
const element = <img src={icon.url}></img>;
Here, the src attribute for the img tag is using a value. We inject this value using expression within a parantheses.
Note here that we didn’t had to use quotes to surround the curly braces.
Conclusion
So we have seen many different ways in which we can utilize React JSX while building React applications. Though it is not mandatory to use JSX with React but in my view, it is the best approach to build React applications.
0 Comments